Actually, I already tried to make one that runs in like linear time but I ran into a few snags. Now that I think about it, all I'm really doing is parsing SB code and coloring it accordingly. I'm not sure if reverse-engineering a program that also does that would be any quicker for me than for me to just make one.
But then again, I'm not sure how to efficiently make one anyway. Sigghhhhhh
This seems kind of confusing. Forgive me if I'm wrong, but if someone made a syntax highlighter, I'm pretty sure they would also have a text editor to go along with it. You might need to make your own to fit your own text editor.
I can try to help, if you want. What do you have so far?
Finished the highlighter. It was actually easier than I first thought, thanks for the help guys.
But uh, seems a bit slow to render at every frame (way too slow). I'll have to come up with some clever way to store and dynamically affect the highlight data.
Edit: aaannd shoot. It's not gonna happen. Even preliminary testing shows me that some kind of syntax highlight data storage isn't going to cut it. Not for the performance I want out of the editor itself. Terrible performance is not worth the cosmetics.
I'll post the highlighter code somewhere though, so I don't feel it went to total waste lol.
Hack together some cheap "multitasking" in your main loop to spread the parsing work across multiple frames, and only reparse/redraw what is visible.
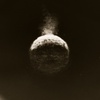
Hmm thought of a better way to store the data and edit the data (at the expense of something else, of course v:v). I feel torn on this, but, like, the numbers don't lie. I should be able to do it. However, it could potentially be cosmetically jarring. Like, suppose you backspace on a line. Depending on the length of that line, it could be anywhere from a second to like 30 seconds to like a minute (again, length of a line matters). Now, as that line is processing, you scroll somewhere else and fumble a few more lines. Oops, accidentally like idk added and then removed a char on a line like +1000 chars long? Say goodbye to highlighting for a few minutes lol.
Actually, there's ways around that of course. Unless I'm ill, I'll have the thing doing parsing check changed tokens. But still, it *could* be jarring. Ex: copy-pasting, adding a " or ' somewhere. Anything that changes the highlight state of large numbers of tokens.
(Unless I treat those cases separately, like, a simple flag or something. For the seamlessness. Gahh, damnit. I'm going to stop for the night.)